This article is about how you can make visible and invisible your layer with the help of checkbox on any base map. The base layer used here is OSM (Open street map), which is open source freely available map. With layers at the same time you can open up multiple data at once and make analysis in view by looking over the overlay layers. Here we will check in Adding Multiple Map Layers – Hide Show layer using leaflet js. You may look over Getting started with leaflet js if you are very new to Leaflet and mapping library.
Adding Multiple Map Layers – Hide Show layer using Leaflet js
Showing multiple layers and making it interactive with hide and show functionality we need checkbox. So, To show two checkboxes on any HTML page the following lines are given. Here checkboxes are taken inside a division, which is denoted by tag <div> and checkboxes take input from user so they define in tag <input>. Every element of HTML can have an id, here we assign for div it is division and for checkboxes it is subunits and places. Outside the tag of checkbox we write name of the checkbox here subunits and places have used.
Some styling is required when it comes to visualisation. In this article the division containing check boxes is shown in the topmost right location. For styling the html element we need to use CSS (cascade sheet style). Using CSS we can define position, background colour font size etc. The z-index property specifies the stack order of an element as two divisions are being used in this article. An element with greater stack order is always in front of an element with a lower stack order. The division of map has lower z index then division of checkboxes.
<body>
<div id=”division” style=”position: absolute; background-color: white; width: 10%; height:10%; float: right; margin: 0.5cm 0cm 0cm 36cm; z-index:999;”>
<input type=”checkbox” id=”subunits” style=”font-size: larger”>Subunits<br>
<input type=”checkbox” id=”places” style=”font-size: larger” > Places
</div>
</body>
Using HTML and CSS we only define how the elements are going to look on the browser. How they will work it is define by the JavaScript or jQuery. Here JQuery has used for checkboxes to work.
Basic syntax is: $(selector).action()
- A $ sign to define/access jQuery
- A (selector) to query HTML elements
- A jQuery action() to be performed on the element.
Here how the code looks like for Adding Multiple Map Layers – Hide Show layer using leaflet js
Before going through code in adding and hide – show multiple layer using leaflet, you should be having a good knowledge of adding point, line and polygon layer using leaflet js along with parsing geojson or topojson layer using leaflet js if you want to add topojson data layers.
The jQuery #id selector uses the id attribute of an HTML tag to find the specific element. Here user needs to click on the checkbox to add and hide the layer from OSM. So selector is id of checkbox and action is click() method. In the method we define the function to add or hide the layer.
$(“#subunits”).click(function() {
if (this.checked) {
console.log(“checked!”);
geo.addTo(newMap);
} else {
console.log(“unchecked!”);
newMap.removeLayer(geo); }; });
In similar way places are added on the map with following lines of code.
$(“#places”).click(function() {
if (this.checked) {
console.log(“checked!”);
var places = topojson.feature(uk,uk.objects.places);
geoP=L.geoJSON(places).addTo(newMap);
} else {
console.log(“unchecked!”);
newMap.removeLayer(geoP);
};
});
Here is how the output will look like for Adding Multiple Map Layers – Hide Show layer using Leaflet js:
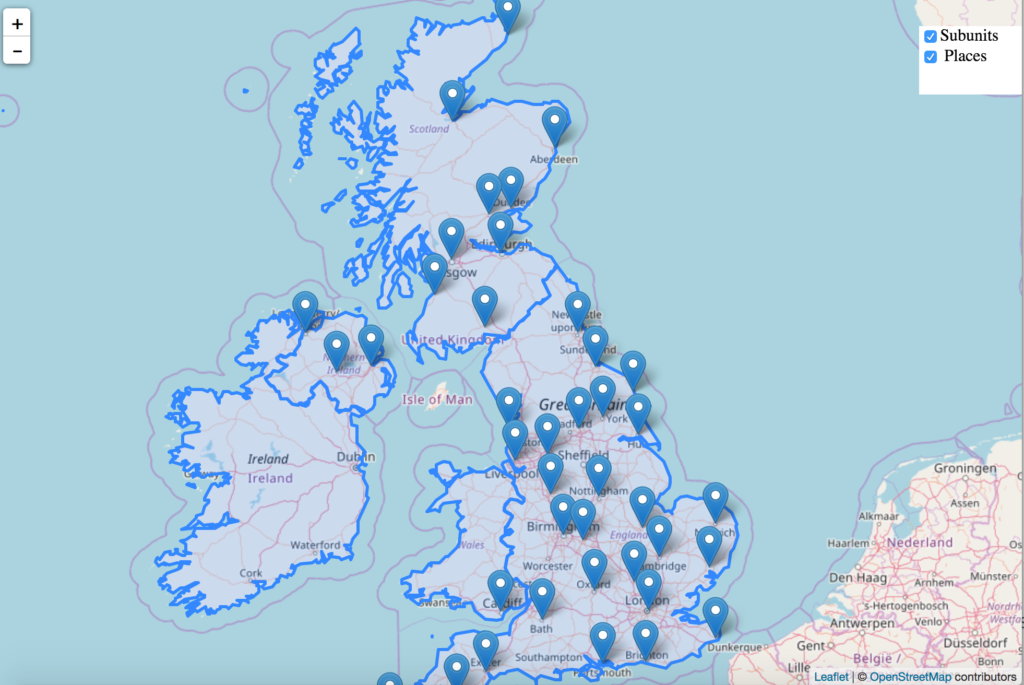
If else statement shows that if checkbox is clicked and returns checked status then layer is added to map and checked can be seen on console. Else checkbox will return unchecked status then unchecked will be written on console and added layer will be removed from map.
Here addTo() and removeLayer() methods are from leaflet javascript library, which adds and removes layer from map. To see the console on browser right click on browser and click to inspect option.
Hope this article might help you in Adding Multiple Map Layer using Leaflet js library. If you are facing any problem in implementing, then do comment below with the problem text you are facing. So that we can discuss over it.